Lugaru's Epsilon Programmer's Editor 14.04
Context:
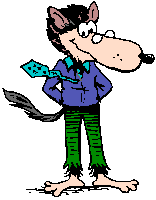
|
Previous
|
Up
|
Next
|
Manipulating File Names |
Primitives and EEL Subroutines |
Parsing URLs |
Epsilon User's Manual and Reference >
Primitives and EEL Subroutines >
File Primitives >
Internet Primitives
int telnet_host(char *host, int port, char *buf)
telnet_send(int id, char *text)
do_telnet(char *host, int port, char *buf)
buffer int telnet_id;
int telnet_server_echoes(int id)
Epsilon provides various commands that use Internet FTP, Telnet and
similar protocols. This section documents how some parts of this
interface work.
All Internet-related functions that retrieve text in the background
and insert it in a buffer do so at the buffer's type_point, just
like Epsilon's process functions.
First, Epsilon provides the primitives telnet_host( ) and
telnet_send( ) for use with the Telnet protocol. The
telnet_host( ) function establishes a connection to a host on the
specified port, and using the indicated buffer. It returns an
identification code. The telnet_send( ) function can use this
code to send text to the host. To kill the telnet job, call
telnet_send( ) and pass NULL as the text. Commands normally call
the telnet_host( ) function through the do_telnet( )
subroutine, which records the telnet identification code in the
buffer-specific telnet_id variable, and does other
housekeeping tasks.
The telnet_server_echoes( ) primitive accepts a telnet
identification code as above, and returns 1 if the server on that
connection is currently set to echo characters sent to it, or 0 if
it is not.
int finger_user(char *user, char *host, char *buf)
int http_retrieve(char *resource, char *host, int port,
char *auth, char *buf, int flags)
char *http_force_headers;
int download_file_to_disk(char *url, char *fname, int sz)
show_url(char *url)
try_show_url(char *url)
The finger_user( ) primitive uses the Finger protocol to
retrieve information on a particular user (if the host is running a
Finger server). It takes the user name, the host, and the name of a
buffer in which to put the results.
The http_retrieve( ) primitive uses the HTTP protocol to
retrieve a page from a web site. It takes a resource name (the final
part of a URL), a host, port, an authorization string (for
password-protected pages) and destination buffer name, plus a set of
flags. The HTTP_RETRIEVE_WAIT flag tells the function not
to return until the transfer is complete. Without this flag the
function begins the transfer and lets it continue in the background.
The HTTP_RETRIEVE_ONLY_HEADER flag tells the function to
retrieve only the header of the web page, not the body. Without this
flag Epsilon will retrieve both; the first blank line retrieved
separates the two.
If the http_force_headers variable is non-null and
non-empty, http_retrieve( ) uses its contents for the HTTP request
it sends to the remote system. It should contain a complete, valid
HTTP request. But if it starts with a + character, then Epsilon
simply adds the rest of its contents to each HTTP request. It should
contain a series of header lines, each terminated by \r\n.
The download_file_to_disk( ) subroutine retrieves the
document from the specified url , then writes it to the disk file
named fname . It returns 0 on success, 1 if retrieving the
file failed, or an error code from writing the file. (A 404 or other
numeric error when retrieving a web page is treated as a success, as
long as it returns a page of some sort. Check the HTTP Headers buffer
for the error code if needed.) The sz parameter should be the
file's expected size; if nonzero, the subroutine displays progress
messages.
The show_url( ) subroutine displays the specified URL in a
web browser, aborting with an error if it couldn't. The similar
try_show_url( ) subroutine displays the URL in a browser,
returning zero if it couldn't, nonzero if it could. A success code
indicates merely that Epsilon was able to find a browser, not that the
specified page exists.
int is_remote_buffer(int buf)
buffer char *buffer_url;
The is_remote_buffer( ) subroutine returns nonzero if the
specified buffer has a telnet or ssh session running, or whose file
name refers to a remote file (one accessed via scp or ftp).
In buffers with a telnet or ssh session, Epsilon sets the
buffer-specific buffer_url variable to the URL used to
create it. This is so it can restart the session later if necessary.
int ftp_op(char *buf, char *log, char *host, int port,
char *usr, char *pwd, char *file, int op)
int do_ftp_op(char *buf, char *host, char *port,
char *usr, char *pwd, char *file, int op)
The ftp_op( ) primitive uses the FTP protocol
to send or retrieve files or get directory listings. It takes the
destination or source buffer name, the name of a log buffer, a host
computer name and port number, a user name and password, a file name,
and an operation code that indicates what function it should perform
(see below).
The do_ftp_op( ) subroutine is similar to ftp_op( ), but
it chooses the name of an appropriate FTP Log buffer, instead of
taking the name of one as a parameter. Also, it arranges for the
appropriate ftp_activity( ) function (see below) to be called,
arranges for character-coloring the log buffer, and initializes the
ftp_job structure that Epsilon uses to keep track of each FTP job.
The FTP_RECV operation code retrieves the specified file
and the FTP_SEND code writes the buffer to the specified
file name. The FTP_LIST code retrieves a file listing from
the host of files matching the specified file pattern or directory
name. The FTP_MISC code indicates that the file name
actually contains a series of raw FTP commands to execute after
connecting and logging in, separated by newline characters. Epsilon
will execute the commands one at a time.
You can combine one of the above codes with some bit flags that
modify the operation. Use the FTP_OP_MASK macro to mask
off the bit flags below and extract one of the operation codes above.
Normally ftp_op( ) returns immediately, and each of these
operations is carried out in the background. Add the code
FTP_WAIT to any of the above codes, and the subroutine will
not return until the operation completes.
The FTP_ASCII bit flag modifies the FTP_RECV and
FTP_SEND operations. It tells Epsilon to perform the transfer
in ASCII mode. By default, all FTP operations use binary mode, and
Epsilon performs any needed line translation itself. But this
doesn't work on some host systems (VMS systems, for example). See
the ftp-ascii-transfers variable for more information.
The FTP_USE_CWD bit flag modifies how Epsilon uses the file
name provided for operations like FTP_RECV, FTP_SEND, and
FTP_LIST. By default, Epsilon sends the file name to the host
as-is. For example, if you try to read a file
dirname/another/myfile , Epsilon sends an FTP command like RETR
dirname/another/myfile . Some hosts (such as VMS) use a different
format for directory names than Epsilon's dired directory
editor understands. So with this flag, Epsilon breaks a file name
apart, and translates a request to read a file such as
dirname/another/myfile into a series of commands to change
directories to dirname , then to another , and then to retrieve
the file myfile . The FTP_PLAIN_LIST bit flag makes
FTP_LIST operations send a LIST command; without it, they
send a LIST -a command so the remote system includes hidden files.
The ftp-compatible-dirs variable controls these bits.
int url_operation(char *file, int op)
The url_operation( ) subroutine parses a URL and begins an
Internet operation with it. It takes the URL and an operation code as
described above for ftp_op( ). If the code is FTP_RECV, then
the URL may indicate a service type of telnet://, http://, or ftp://,
but if the code is FTP_SEND or FTP_LIST, the service type
must be ftp://. It can modify the passed URL in place to put it in a
standard form. It calls one of the functions do_ftp_op( ),
http_retrieve( ), or do_telnet( ) to do its work. It returns
0 on success. On failure it either returns nonzero or aborts,
depending on the type of error.
ftp_misc_operation(char *url, char *cmd)
The ftp_misc_operation( ) subroutine uses the
do_ftp_op( ) subroutine to perform a series of raw FTP commands.
It takes an ftp:// URL (ignoring the file name part of it) connects
to the host, logs in, and then executes each of the newline-separated
FTP commands in cmd . Dired uses this function to delete or move
a group of files.
buffer int (*when_net_activity)();
net_activity(int activity, int buf, int from, int to)
As Epsilon performs Internet functions, it calls an EEL function to
advise it of its progress. The buffer-specific variable
when_net_activity contains a function pointer to the
function to call. Epsilon uses the value of this variable in the
destination buffer (or, in the case of the NET_LOG_WRITE and
NET_LOG_DONE codes below, the log buffer). If the variable is
zero in a buffer, Epsilon won't call any EEL function as it proceeds.
The EEL function will always be called from within a call to
getkey( ) or delay( ), so it must save any state information
it needs to change, such as the current buffer, the position of
point, and so forth, using save_var . The subroutine
net_activity() shown above indicates what parameters the function
should take--there's not actually a function by that name.
The activity parameter indicates the event that just occurred. A
value of NET_RECV indicates that Epsilon has just received
some characters and inserted them in a buffer. The buf parameter
tells which buffer is involved. The from and to values
indicate the new characters. A value of NET_DONE means
that the net job running in buffer buf has finished. The above
are the only activity codes generated for HTTP, Telnet, or Finger
jobs.
FTP jobs have some more possible codes. NET_SEND indicates
that another block of text has been sent. In this case, from
indicates that number of bytes sent already from buffer buf , and
to indicates the total number of bytes to be sent. The code
NET_LOG_WRITE indicates that some more text has been
written to the log buffer buf , in the range from ...to .
Finally, the code NET_LOG_DONE indicates that the FTP
operation has finished writing to the log buffer. It occurs right
after a NET_DONE call on FTP jobs.
ftp_activity(int activity, int buf, int from, int to)
finger_activity(int activity, int buf, int from, int to)
telnet_activity(int activity, int buf, int from, int to)
buffer int (*buffer_ftp_activity)();
The file epsnet.e defines the when_net_activity functions shown
above, which provide status messages and similar things for each type
of job. The ftp_activity( ) subroutine also calls a
subroutine itself, defined just like these functions, through the
buffer-specific variable buffer_ftp_activity . The
dired command uses this to arrange for normal FTP activity
processing when retrieving directory listings, but also some
processing unique to dired.
int gethostname(char *host, ?int method)
The gethostname( ) primitive
sets host to the computer's host name and returns 0 . If it
can't for any reason, it returns 1 and sets host to "?".
The method parameter controls which type of host name Epsilon
retrieves. By default, it uses the machine's locally-configured host
name. A value of 3 makes it instead retrieve the host's fully
qualified domain name using DNS. Values of 1 or 2 make it do
this only under Windows or Unix, respectively. Retrieving the DNS
name in some network configurations can cause on-demand auto-dialing
or delays if the machine's DNS server isn't accessible.
Previous
|
Up
|
Next
|
Manipulating File Names |
Primitives and EEL Subroutines |
Parsing URLs |

Epsilon Programmer's Editor 14.04 manual. Copyright (C) 1984, 2021 by Lugaru Software Ltd. All rights reserved.
|