Lugaru's Epsilon Programmer's Editor 14.04
Context:
| Window Titles and Mode Lines
|
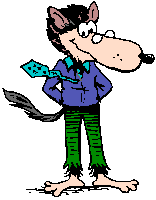
|
Previous
|
Up
|
Next
|
Buffer Text in Windows |
Primitives and EEL Subroutines |
Normal Buffer Display |
Epsilon User's Manual and Reference >
Primitives and EEL Subroutines >
Display Primitives >
Window Titles and Mode Lines
window_title(int win, int edge, int pos, char *title)
#define TITLECENTER (0)
#define TITLELEFT(offset) (1 + (offset))
#define TITLERIGHT(offset) (-(1 + (offset)))
make_title(char *result, char *title, int room)
You can position a title
on the top or bottom border of a window using the
window_title( ) primitive. (Also see the
set_window_caption( ) primitive described in Windowed Dialogs.) It takes the window number in win
and the text to display in title . (It makes a copy of the text,
so you don't need to make sure it stays around after your function
returns.) The edge parameter must have the value of BTOP
or BBOTTOM, depending on whether you want the title displayed
on the top or bottom border of the window.
Construct the pos parameter using one of the macros
TITLELEFT() , TITLECENTER , or
TITLERIGHT() . The TITLECENTER macro centers the
title in the window. The other two take a number which says how many
characters away from the given border the title should appear. For
example, TITLERIGHT(3) puts the title three characters away from
the right-hand edge of the window.
Epsilon interprets the percent character "%" specially
when it appears in the title of a window. Follow the percent
character with a character from the following list, and Epsilon will
substitute the indicated value for that sequence:
- %c
- Epsilon substitutes the current column number, counting
columns from 0.
- %C
- Epsilon substitutes the current column number, counting
columns from 1.
- %d
- Epsilon substitutes the current display column, with a
< before it, and a space after. However, if the display column
has a value of 0 (meaning horizontal scrolling is enabled, but
the window has not been scrolled), or -1 (meaning the window
wraps long lines), Epsilon substitutes nothing.
- %D
- Epsilon substitutes the current display column, but if the
display column is
-1 , Epsilon substitutes nothing.
- %l
- Epsilon substitutes the current line number.
- %m
- Epsilon substitutes the text "
More ", but only if
characters exist past the end of the window. If the last
character in the buffer appears in the window, Epsilon substitutes
nothing.
- %P
- Epsilon substitutes the percentage of point through the
buffer, followed by a percent sign.
- %p
- Epsilon substitutes the percentage of point through the
buffer, followed by a percent sign. However, if the bottom of the
buffer appears in the window, Epsilon displays Bot instead. Epsilon
displays Top if the top of the buffer appears, and All if the entire
buffer is visible.
- %s
- Epsilon substitutes "
* " if the buffer's modified
flag has a nonzero value, otherwise nothing.
- %S
- Epsilon substitutes "
* " if the buffer's modified
flag has a nonzero value, otherwise nothing.
- %h
- Epsilon substitutes the current hour in the range 1 to 12.
- %H
- Epsilon substitutes the current hour in military time in the
range 0 to 23.
- %n
- Epsilon substitutes the current minute in the range 0 to 59.
- %e
- Epsilon substitutes the current second in the range 0 to 59.
- %a
- Epsilon substitutes "am" or "pm" as appropriate.
- Note:
- For the current time, use a sequence like
%2h:%02n %a for "3:45 pm" or %02H:%02n:%02e for "15:45:21".
- %%
- Epsilon substitutes a literal "%" character.
- %
< - Indicates that redisplay may omit text to the left,
if all of the information will not fit.
- %
> - Puts any following text as far to the right as possible.
With any of the numeric sequences, you can include a printf-style
field width specifier between the % and the letter. You can use
the same kinds of field width specifiers as C's printf()
function. In column 9, for example, the sequence %4c
expands to " 9 ", %04c expands to "0009 ",
and %-4c expands to "9 ".
You can expand title text in the same way as displaying it would,
using the make_title( ) primitive. It takes the title to
expand, a character array where it will put the resulting text, and
a width in which the title must fit. It returns the actual length of
the expanded text.
prepare_windows() /* disp.e */
window char _window_flags;
#define FORCE_MODE_LINE 1
#define NO_MODE_LINE 2
#define WANT_MODE_LINE 4
build_mode() /* disp.e */
assemble_mode_line(char *line) /* disp.e */
set_mode(char *mode) /* disp.e */
buffer char *major_mode; /* EEL variable */
user char mode_format[60];
clean_mode(char *mode)
Whenever Epsilon thinks a window's mode line or title may be out of
date, it arranges to call the prepare_windows( ) and
build_mode( ) subroutines during the next redisplay. The
prepare_windows( ) subroutine arranges for the correct sort of
borders on each window. This sometimes depends on the presence of
other windows. For example, tiled windows get a right-hand border
only if there's another window to their right. This subroutine will
be called before text is displayed.
By default, prepare_windows( ) puts a mode line on all tiled
windows, but not on any pop-up windows. You can set flags in the
window-specific _window_flags variable to change this. Set
FORCE_MODE_LINE if you want to put a mode line on a pop-up
window, or set NO_MODE_LINE to suppress a tiled window's mode
line. The prepare_windows( ) subroutine interprets these flags,
and alters the WANT_MODE_LINE flag to tell build_mode( )
whether or not to put a mode line on the window.
The build_mode( ) subroutine calls the
assemble_mode_line( ) subroutine to construct a mode line, and
then uses the window_title( ) primitive to install it.
The assemble_mode_line( ) subroutine calls the set_mode( )
subroutine to construct the part of the mode line between square
brackets (the name of the current major mode and a list of minor
modes).
While many changes to the mode line require a knowledge of EEL, you
can do some simple customizations by setting the variable
mode_format. Edit these variables with set-variable,
using the percent character sequences listed above. For example, if
you wanted each mode line to start with a line and column number, you
could add the text " Line %l Col %c " to mode_format.
An EEL function can add text to the start of a particular buffer's
mode line by setting the buffer-specific variable mode_extra.
Call the set_mode_message( ) subroutine to do this. It
takes a pointer to the new text, or NULL to remove the current
buffer's extra text. Internet FTPs use this to display the percent
of a file that's been received (and similar data).
The set_mode( ) subroutine gets the name of the major
mode from the buffer-specific major_mode variable, and adds
the names of minor modes after it.
You can add new
minor modes by defining a function with a name that starts with
show_minor_mode_ . It must take one parameter, a character
pointer. When called, it should copy the minor mode name to the
character pointer if the mode is in effect.
Sometimes Epsilon constructs variable or function names that include
the current mode's name, to permit a mode to define its own value for
some function. For instance, saving a file looks for a variable named
modename-add-final-newline , where modename is the
current mode's name. Since the mode name may contain characters that
aren't valid in a variable name, functions can call the
clean_mode( ) subroutine to get a version of the major mode
with any invalid characters removed. Only (lowercased) alphanumerics,
_ and - (converted to _ ) will be copied to mode .
display_more_msg(int win)
The display_more_msg( ) subroutine makes the bottom border of
the window win display a "More" message when there are
characters past the end of the window, by defining a window title
that uses the %m sequence.
Previous
|
Up
|
Next
|
Buffer Text in Windows |
Primitives and EEL Subroutines |
Normal Buffer Display |

Epsilon Programmer's Editor 14.04 manual. Copyright (C) 1984, 2021 by Lugaru Software Ltd. All rights reserved.
|